Contact us : contact@digitechgenai.com
Introduction
In web development, Vue.js has become a widely used JavaScript framework for developing scalable and strong applications. Its simplicity, flexibility, and strong ecosystem make it a developer’s favorite. In this article, we will discuss the architecture of Vue.js, its major components, structure, and best practices.
What is Vue.js Architecture?
Vue.js architecture means the structure and organization of a Vue.js application. It involves how components are structured, templates are rendered, and directives manipulate the DOM. Properly organized Vue.js architecture is crucial in developing maintainable, efficient, and scalable applications.
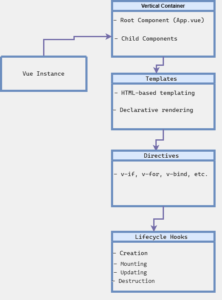
Important Elements of Vue.js Structure
1. Vue Instance
The Vue instance is the central part of a Vue.js application. It is the root of the component tree, and other components are nested inside it. The Vue instance is in charge of controlling the state of the application, rendering templates, and processing user input.
// main.js
import Vue from 'vue'
import App from './App.vue'
new Vue({
render: h => h(App)
}).$mount('#app')
2. Components
Components are reusable chunks of code that constitute part of the UI of an application. Components can be nested within one another to create a compound component tree. Vue.js components have a template, script, and style block.
<!-- HelloWorld.vue -->
<template>
<div>{{ message }}</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello, World!'
}
}
}
</script>
<style scoped>
div {
color: #42b983;
}
</style>
3. Templates
Templates are declarative and based on HTML, with declarative rendering being used to bind data to the DOM. Vue.js templates rely on a virtual DOM, which helps render and update components efficiently.
<!-- template.html -->
<template>
<div>
<p>{{ title }}</p>
<ul>
<li v-for="item in items" :key="item.id">{{ item.name }}</li>
</ul>
</div>
</template>
4. Directives
Directives are unique properties prefixed with v-. They’re employed to modify the DOM, conditionally render components, and bind data to components.
<!-- directive.html -->
<template>
<div>
<p v-if="showMessage">{{ message }}</p>
<button v-on:click="toggleMessage">Toggle Message</button>
</div>
</template>
5. Lifecycle Hooks
Lifecycle hooks are methods called at various stages in a component’s life cycle. They offer a means of running code while creating, mounting, updating, and destroying components.
// lifecycle-hooks.js
export default {
beforeCreate() {
console.log('Before Create')
},
created() {
console.log('Created')
},
beforeMount() {
console.log('Before Mount')
},
mounted() {
console.log('Mounted')
}
}
Best Practices for Vue.js Architecture
- Make Components Small and Focused: Every component should have one responsibility and be reusable.
- Use a Consistent Naming Convention: Use a consistent naming convention for components, props, and events.
- Use Vuex for State Management: Use Vuex to handle global state and prevent passing props down several levels.
- Use Vue Router for Client-Side Routing: Use Vue Router to handle client-side routing and navigation.
Conclusion
In summary, Vue.js architecture is an essential factor in developing strong and scalable web applications. By learning the core elements, architecture, and best practices of Vue.js, developers can develop maintainable, efficient, and scalable applications. With its simplicity, flexibility, and strong ecosystem, Vue.js has become a top choice for web development. Following the best practices discussed in this article, you can elevate your Vue.js development to the next level and develop applications that are fast, efficient, and scalable.
Read more related blogs: Vue.js
FAQs
Q1: What is Vue.js architecture, and why is it important?
Vue.js architecture refers to the organization and structure of a Vue.js application. It’s essential for building maintainable, efficient, and scalable applications. A well-structured Vue.js architecture helps developers to efficiently develop and manage complex applications.
Q2: What are the key components of a Vue.js application?
The key components of a Vue.js application include
- Vue Instance: The core of a Vue.js application, responsible for managing the application’s state and rendering templates.
- Components: Reusable pieces of code that represent a part of the application’s UI.
- Templates: HTML-based templates that use declarative rendering to bind data to the DOM.
- Directives: Special attributes that begin with the
v-
prefix, used to manipulate the DOM.
Q3: How do I structure my Vue.js project for scalability and maintainability?
To structure your Vue.js project for scalability and maintainability, follow these best practices
- Use a standard file structure: Stick with the file structure generated by the Vue CLI, and add custom directories and files as needed.
- Use Single-File Components: Define each component in its own dedicated file, using the
.vue
extension. - Use PascalCase for component names: Name your components in PascalCase, and use a consistent prefix for base components.
Q4: What is the difference between Options API and Composition API in Vue.js?
The Options API and Composition API are two different ways to author Vue.js components. The Options API uses an object of options to define a component’s logic, while the Composition API uses imported API functions to define a component’s logic
Q5: How do I manage state in a Vue.js application?
You can manage state in a Vue.js application using Vuex or Pinia, which are state management libraries that provide a centralized store for your application’s state
Q6: What are lifecycle hooks in Vue.js, and how do I use them?
Lifecycle hooks are functions that are called at different stages of a component’s lifecycle. You can use lifecycle hooks to execute code during the creation, mounting, updating, and destruction of components.
Q7: How do I optimize the performance of my Vue.js application?
To optimize the performance of your Vue.js application, use techniques like code splitting, lazy loading, and caching. You can also use Vue’s built-in features like computed properties and memoization to improve performance.
Q8: What are some common pitfalls to avoid when building a Vue.js application?
Some common pitfalls to avoid when building a Vue.js application include:
- Tight coupling: Avoid tightly coupling components to each other or to the global state.
- Not using a standard file structure: Use a consistent file structure to make your code more maintainable.
- Not optimizing performance: Use techniques like code splitting and lazy loading to improve performance.
Q9: How do I integrate Vue.js with other libraries and frameworks?
You can integrate Vue.js with other libraries and frameworks by using plugins, mixins, or wrapper components. Some popular integrations include Vue Router, Vuex, and Vuetify.
Q10: What are some best practices for building scalable and maintainable Vue.js applications?
Some best practices for building scalable and maintainable Vue.js applications include:.
- Use a standard file structure: Stick with the file structure generated by the Vue CLI.
- Use Single-File Components: Define each component in its own dedicated file.
- Use consistent naming conventions: Use PascalCase for component names and consistent prefixes for base components.
Recommended Resources: